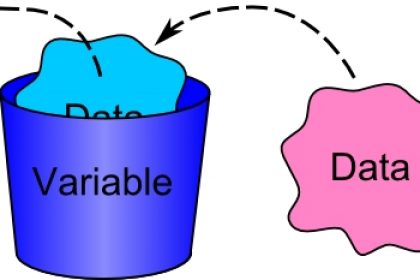
Having covered a basic introduction in Java Programming language and structure of a typical Java Application in the previous blogs, this blog takes a deeper look into Java variables, their types and use.
What is a variable?
A variable is a named memory location that holds a value while a program is executed.
Types of Variables
Java has three types of variables based on the scope and the location within the program. These are: local, instance and static variables.
Local Variables. Are variables declared inside the body of a method? Local variables are only visible to the methods in which they are declared; they are not accessible from the rest of the class. There is no special keyword designating a variable as local and the static keyword cannot be used on them.
Instance Variables (Non-Static Fields). This is a field declared inside the class but outside the body of the method. It is not declared as static. A non-static variable contains values that are unique to each instance (object) of a class and is not shared among instances.
Class Variables (Static Fields). A class variable is any field declared with the static modifier. This means that only a single copy of static variable is created and is shared among all the instances (objects) of the class.
For more information about variables in java visit https://docs.oracle.com/javase/tutorial/java/nutsandbolts/variables.html
– – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – –
LIVE A LITTLE
Why did the WiFi and the computer get married?
Because they had a connection.
– – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – –
Using a quadratic equation, we shall try to calculate the roots of a quadratic equation to give a clear picture of how variables are used in java.
Write the following code:
package quadratic
import java.util.Scanner;
public class Quadratic {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Input a: ");
double a = input.nextDouble();
System.out.print("Input b: ");
double b = input.nextDouble();
System.out.print("Input c: ");
double c = input.nextDouble();
double root1 = ((-b) + Math.sqrt(b * b - (4 * a * c))) / 2 * a;
System.out.println("The value of root1 is: " + root1);
double root2 = ((-b) - Math.sqrt(b * b - (4 * a * c))) / 2 * a;
System.out.println("The value of root2 is: " + root2);
}
}
Understanding the code:
//This invokes a package “Scanner” that allows user input when prompted
import java.util.Scanner;
/*Create an object of a “Scanner” class which is define in import “java.util.Scanner” package. Scanner class allows user to take input from console.*/
Scanner input = new Scanner(System.in);
//Prompt user for terms a, b and c respectively and store them in variables of data type “double”:
System.out.print("Input a: ");
double a = input.nextDouble();
System.out.print("Input b: ");
double b = input.nextDouble();
System.out.print("Input c: ");
double c = input.nextDouble();
//Quadratic formula that derives root1
//“Math.sqrt” invokes a square root function that affect the formula inside its parenthesis, that is “(b * b - (4 * a * c)).”
double root1 = ((-b) + Math.sqrt(b * b - (4 * a * c))) / 2 * a;
//Displays the result obtained for root1
System.out.println("The value of root1 is: " + root1);
//Quadratic formula that derives root2
double root2 = ((-b) - Math.sqrt(b * b - (4 * a * c))) / 2 * a;
//Displays the result obtained for root2
System.out.println("The value of root2 is: " + root2);
If you want a concise path to conquering variables, here’s the steps to help you achieve that:
- Defining variables
- Identifying types of variables
- Identifying variable naming rules and conventions
- Assigning and declaring variables
- Identifying data types
- Writing a quadratic application to illustrate the concepts of the blog
- Explaining the application code
To realize the full potential of variables, there is need to understand how to use them in mathematical expressions and the different operations that can be performed on them. The possibilities are endless! Stay tuned.