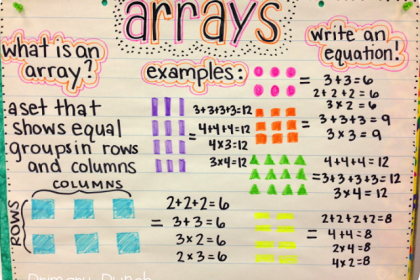
– – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – –
LIVE A LITTLE:
A woman and her husband were having a conversation about her boss…
Woman:My boss wants to sleep with me.
Man:What!! and why? Don’t you dare think about it!
Woman:But he has an offer! He says he will drop 2,500 dollars on the ground and by the time I pick it up, he will be done.
Man: That’s quite a lot of money. We could really use it! Here’s what you’ll do when he drops it, pick it up quickly and tell him you are going home to your husband.
A few hours later…..
Man:Were you able to do it?
Woman:That bastard used coins!!
– – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – –
So in the previous lesson we learned about classes (refer to blog on classes using a JSON string) in Java and we used the student class. In this blog using the same concept, we shall register a group of students using an array and use the help of a list. We shall be able to see the list of the students.
Refer to the blog Class in java
Compile the ArrayStudent class since it contains the main class.
Output:
Use the same student class and the class below for list to solve the same problem.
Compile the ListStudent class since it contains the main class.
Output:
Explanation:
This program requests and takes the name, age and sex of a student, creates a student object parses the object for the attributes and stores them in an array, then retrieves and displays them from the array
// This imports the scanner package to take in user input
import java.util.Scanner;
// here we create class student
public class Student {
//Then we declare variables to be used in the program that represent the student. Public to be accessed from anyway in the program.
Public String name //student first name
public String age; //Student Age
public String sex; //Student Gender
//Setter and Getter Methods for the Student Class Attributes
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setAge(String age) {
this.age = age;
}
public String getAge() {
return age;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getSex() {
return sex;
}
//Student Class Constructor
public Student() {
Scanner n = new Scanner(System.in);
System.out.print(“First Name: “);
this.setName(n.next());
System.out.print(“Age: “);
this.setAge(n.next());
System.out.print(“Gender: “);
this.setSex(n.next());
}//End of constructor
}//End of Student class
This is the class that contains the main method and it uses arrays.
//Start of array class
public class ArrayStudent {
//main method
public static void main(String[] args) {
//Create Array to store Students
Student[] studentArray = new Student[3];
//Add Students to Array
for (int i=0; i<3; i++) {
Student s = new Student();
studentArray[i] = s;
}//End of for loop
//Display Students from Array
for(int i=0; i<3; i++) {
Student s = studentArray[i];
System.out.println(s.getName()+”, “+s.getAge()+”, “+s.getSex());
}End of the for loop
}End of main method
}End of array class
This is the class that contains the main method and it uses list.
// This imports the ArrayList package to take in user input
import java.util.ArrayList;
// This imports the List package to take in user input
import java.util.List;
//Start of ListStudent class
public class ListStudent {
//Main method
public static void main(String[] args) {
//Create List to store Students
List<Student> studentList = new ArrayList<Student>();
//Add Students to List
Student s1 = new Student();
studentList.add(s1);
Student s2 = new Student();
studentList.add(s2);
Student s3 = new Student();
studentList.add(s3);
//Display Students from List
for(int i=0; i<3; i++) {
Student s = studentList.get(i);
System.out.println(s.getName()+”, “+s.getAge()+”, “+s.getSex());
}//End of for loop
}//End of main method
}//End of list class