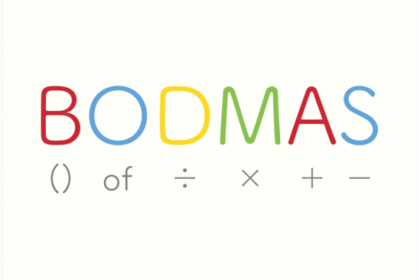
We shall continue from where we left off last time on Java Programming. Have you heard of mathematical expressions before? Well, if you haven’t we will have a clear understanding of what they are and how to solve them, not just by using a calculator but also using a Java application.
An operation on two numbers is easy, but how do we solve an expression with brackets and multiple operations? It is not as simple as an expression involving two numbers.
Let’s consider the BODMAS rule and learn about simplification of brackets.
Brackets
Of
Division
Multiplication
Addition
Subtraction
This means that we evaluate our expression starting with brackets first then other operations will follow according to the order of precedence. In this java program we shall compute an expression following the BODMAS rule. Consider an expression such as
7+12/ (2*3)-4
How does a computer program evaluate the following expression? Does it start off from right to left? Or does it start from left to right?
The evaluation of this expression is as follows:
7+12/ (6)-4
7+2-4
9-4
5
LIVE A LITTLE…
————————————————————————————————-
A Rasta man went to the bank with a sack full of weed…
Teller : How may I help you sir?
The guy puts the sack of weed on the counter
Rasta Man : I want to open a joint account.
————————————————————————————————-
Following the evaluation of the above expression, we shall begin. First, we created a class that does validation to check if the expression is correctly written removing all the spaces in the expression and irrelevant data. In this class we created the following methods;
- //Checks if the character at the beginning of the expression is valid
public static boolean validateFirstindex(){
- //Checks if the end of the expression is valid
public static boolean endExpression () {
- //Check whether all brackets are balanced
public static boolean checkBrackets() {
- //ensures the Expression contains valid characters
public static boolean validCharacters () {
- ensures that two subsequent operators are valid and in the correct order
public static boolean operatorSeq() {
- //Remove spaces from the expression
public static void removeSpaces () {
- //when number is followed by an opening bracket, call this method to insert the multiplication operator
public static void insertMultiplication (int index) {
- //Validates the expression
public static char [] validate (char [] expression) {
Next step, we created a class that does the evaluation of the expression. We used two stacks, one to store operators and the other to store the values. We created different methods which handle specific tasks. Some of the methods used are explained below.
The code below is a snippet of one of the methods that we used to evaluate. You can find the code for the entire program in our GitHub account.
Explanation:
//Stack for numbers: ‘values’
Stack<Double> values = new Stack<Double> ();
// Stack for Operators: ‘ops’
Stack<Character> ops = new Stack<Character> ();
//Declare and Initialize i to 0
int i = 0;
//Loop through the tokens
while ( i < tokens.length ) {
// Current token is a number, push it to stack for numbers
if ((tokens[i] >= ‘0‘ && tokens[i] <= ‘9‘) || tokens[i] == ‘.‘) {
StringBuffer sbuf = new StringBuffer();
// There may be more than one digits in number
int j = i;
while (j < tokens.length) {
if ((tokens[j] >= ‘0‘ && tokens[j] <= ‘9‘) || tokens[j] == ‘.‘){
sbuf.append(tokens[j++]);
}//End of if statement
else break;
}//End of while loop
i = j–1;
values.push(Double.parseDouble(sbuf.toString()));
i++;
}//End of if statement
The other method that we will explain about is the one used to check the operator’s order of precedence.
Explanation:
// Returns true if ‘op2’ has higher or same precedence as ‘op1’, otherwise returns false.
public static boolean hasPrecedence(char op1, char op2) {
if (op2 == ‘(‘ || op2 == ‘)‘){
return false;
}//End of if statement
if ((op1 == ‘&‘ || op1 == ‘*‘ || op1 == ‘/‘) && (op2 == ‘+‘ || op2 == ‘–‘)){
return false;
}//End of if statement
else {
return true;
}//End of else
}//End of method
The last method we will explain is the one used to solve the expression having met all the conditions of a proper expression.
Explanation:
// A utility method to apply an operator ‘op’ on operands ‘a’ and ‘b’. Return the result.
public static double solve(char op, double b, double a) {
switch (op)
{
case ‘+‘:
return a + b;
case ‘–‘:
return a – b;
case ‘*‘:
return a * b;
case ‘&‘:
return a * b;
case ‘/‘:
if (b == 0)
throw new
UnsupportedOperationException(“Cannot divide by zero“);
return a / b;
}//End of switch case
return 0;
}//End of solve method
p { margin-bottom: 0.25cm; direction: ltr; line-height: 115%; text-align: left; }a:link { color: rgb(5, 99, 193); }