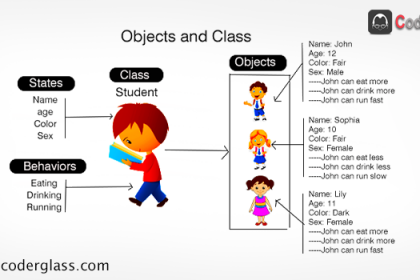
JSON, is not a name of some tall, handsome guy that ladies always stare at or some guy that boys wish to grow into, just in case you are wondering! No, our JSON is an acronym for JavaScript Object Notation. It is a syntax for storing and exchanging data written with JavaScript Object Notation.
It outputs data surrounded by curly braces and quotes separated by commas, making it easily readable by humans and at the same time efficiently parsed by code. Json string, outputs data in continous line.For this code we shall be using JSON raw to explain what classes are and how they are used in java.
Basically a JSON string should appear as;
Example : {“Name”:”Jack”,”Age”:”24”,”Sex”:”Female”}
– – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – –
LIVE A LITTLE:
These two strings walk into a bar and sit down. The bartender says, “So what’ll it be?”
The first string says, “I think I’ll have a beer quag fulk boorg jdk^CjfdLk jk3s d#f67howe%^U r89nvy owmc63^Dz x.xvcu”
“Please excuse my friend,” the second string says, “He isn’t null-terminated.”
– – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – –
Let’s look at the code below of the Student class:
This other code for Project class:
Output:
Explanation:
This is the Student Class which does not have the main class hence cannot be compiled;
//Start of the student class
public class Student {
//Declare variables as public to make them accessible by other classes
public String name;
public String age;
public String sex;
/*Setter and Getter Methods for the Student Class Attributes which are used to effectively protect your data when creating classes.
set() method sets the value followed by the variable name capitalised. e.g setName()
set() method takes a parameter and assigns it to the attribute.
get() method returns its value followed by the variable name capitalized. It returns the value of attribute. it’s written as getName().
*/
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setAge(String age) {
this.age = age;
}
public String getAge() {
return age;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getSex() {
return sex;
}
//Method that returns the value of the Student object as a JSON String
public String getJson() {
//Initializing the Json characters as a String
String brace = “{“;
String close = “}”;
String quote = “\””;
String colon = “:”;
String comma = “,”;
//Creating the Json Raw String
String json = brace + quote + “Name” + quote
+ colon + quote + name + quote
+ comma
+ quote + “Age”+ quote
+ colon + quote + age + quote
+ comma
+ quote + “Sex” + quote + colon + quote + sex + quote + close;
return json;
}//End of getJson method
}//End of Student class
This is the Project class which contains the main method;
import java.util.Scanner;
//Start of the Project class
public class Project {
//start of the main method
public static void main(String []args){
//Call the readStudent method to the main method
Student st1 = readStudent();
System.out.println(st1.getJson());
}//End of main method
//Method to read and return a student object
public static Student readStudent() {
//Call the student class to be used as an object
Student st = new Student();
//Prompts user to enter values for first name, age and gender respectively and stores the value in object st
Scanner n = new Scanner(System.in);
System.out.print(“First Name: “);
//the object st stores the input entered. For this case, the name.
st.setName(n.next());
System.out.print(“Age: “);
st.setAge(n.next());
System.out.print(“Gender: “);
st.setSex(n.next());
System.out.println();
//returns the value of the object
return st;
}//End of readStudent method
}//End of Project Class
We followed the following steps in order to achieve the above task.
- Understanding JSON and how it works.
- Use JSON to understand classes in java and how they are used.
- Understanding and Explaining the code.