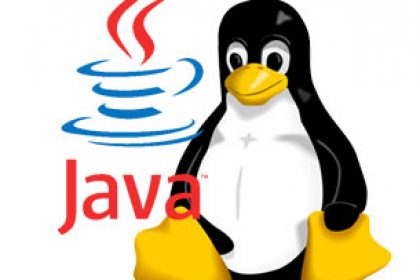
Learning Java in Linux
One of the main motivations for the Java programming language is the notion of having the ability to write one set of source code and give the resulting program the ability to run on different operating systems or operating environments.
Java has this “write once, run anywhere” ability due to the way the compiler translates the source code into a special file called a “Byte Code” file, that can run under any supported Java Runtime Environment (JRE).
Java development process steps ;
- Write the Java source code and save this code in one or more plain text files. These files typically have .java at the end of their file name.
- Run the Java Compiler (javac) to “compile” the source code into a “Byte Code” file. These Byte Code files typically have .class at the end of their file name.
- Run the program by submitting the byte code file to a Java Runtime Environment by way of the java
The advantage of this approach is that it is generally possible to copy the byte code (.class) file to any operating system that supports a JRE, and run the program directly.
Download and Install Java Development Kit
Most Linux distributions such as Debian. Ubuntu, Red Hat and CentOS include a software repository tool that automates the download and install steps. For example, under Debian and Ubuntu Linux distributions, the apt-get command can be used to download and install the JDK. For example:
sudo apt-get update
sudo apt-get install oracle-java12-installer
Writing, Compiling and Testing your first Java Program.
When I started programming in Java, I was lost simply because I did not understand how to build applications using this language. So I decided to build this app as my first Java application. In Linux the nano or pico editors or edit/kedit or kate programs are fine for editing Java source code files. In our case, we will use kate. For the purposes of this series we will stick to using simple tools.
The first program we will write is a very simple “Area of a Rectangle” example.
- In Linux, search for the Terminal program and open it.
- Create a new file using the kate program by searching for it. The kate program will open as follows.
- Type in the following Java source code (we’ll explain what each line does below).
public class Area{
public static void main(String []args){
int length;
int width ;
int area;
length = 4;
width = 3;
area = (length * width);
System.out.println(“The area is “+area);
}
}
- Save your file by clicking on “file” located at the top on your left. A drop down will appear then select “save as”. Name your file with an extension of .java. Make sure the name of your file resembles that of your class. Click save. When completed kate should now look like the following:
As a way of explanation, here are some comments (followed by the //) that describe the program.
– – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – –
LIVE A LITTLE
Why was the computer late for work? Because it had a hard drive
– – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – – –
//This is the start of our program. A public class is accessible by all the java code, even outside the package.
public class Area{
/*The main method where our program begins. Program cannot execute without this method. public – access specifier means from everywhere we can access it static – access modifier means we can call this method directly using class name without creating an object of it void – it returns nothing main – method name string [] args – in java accept only string type of argument and stores it in a string*/
public static void main (String []args){
//Declare your variables of data type integer
int length;
int width;
int area;
//Initialize your variables
length = 4;
width = 3;
//area stores the result after computing (length * width)
area = (length * width);
//Prints the result.
System.out.println (“The area is “+area);
//End of “main” method
}
//End of the class
}
- Exit out of kate.
- Compile the program using the javac Type this command javac Area.java as shown below in the terminal.
- If there are no syntax errors in your code, the compiler will simply finish up silently.
- If Linux cannot locate the javac compiler, the following error message will appear:
No command ‘javac’ found, but there are 18 similar ones
javac: command not found
Solution: Install jdk.
- Now run your sample program by running java followed by the name of your program
as shown below java Area in the terminal.
Now you have an idea on how to write and run a Java program, does being a programmer sound so mystical or scary? guess not. The fun doesn’t end there! Linux is user friendly only that its particular on who its friends are. If you are a Windows user, join the ride in the next post.